Jira provides REST APIs to interact with Jira on both Server and Cloud platforms. Using REST APIs developers can create scripts/programs to access resources such as projects, issues, comments, users etc., from Jira. A resource is some data entity in Jira and to get that resource your script need to send a HTTP request to a specific URI and parse the response that you receive. The URIs have the following structure:
https://<site-url>/rest/api/3/<resource-name>
For example, an HTTP request to following URI gets a list of all the projects.
https://mysite.atlassian.net/rest/api/3/project
The Jira REST API uses standard HTTP methods like GET, PUT, POST and DELETE. The data exchange format used is JSON.
The scripts to access the resources from JIRA can be written in any programming language that allows you to send HTTP requests and can receive and parse the response. Here are some basic examples of how to retrieve data from Jira cloud using Python.
Sending HTTP Requests and parsing the Response
The requests library in Python contains objects and functions that enables you to send and receive requests and response using commonly used HTTP methods. You can also pass a set of key-value pairs as request headers using the headers parameter. Header parameters provide many useful information such as the Content Type, Authorization parameters etc. To use the request library in Python, import the request
module.
import requests
To create and send a request you can either call the request method or the get method. The response the you receive is in JSON format and to parse the response you can make use of the Python json module that provides API to encode and decode JSON string to Python objects and vice versa.
To learn how to read and write JSON data in Python visit:
How to read and write JSON data using Python
User Authentication
To create, view or search issues in a Jira cloud project, users have to be logged in (unless Anonymous access is enabled) and have the right permissions to do so and the same applies to Jira REST API also.
The first step for using REST API therefore is user authentication. There are different ways to authenticate a user:
- using email and API token
- Cookie based authentication
- OAuth 1.0a
- OAuth 2.0 authorization code grants (3LO) for apps (recommended for Apps that doesn't use Atlassian Connect)
- Atlassian Connect libraries for Connect Apps
The examples in this article use email and API token for authentication which is the easiest and recommended method of Basic authentication. In this method, your email address is your user name and the API token will be used as the password.
API Token
To generate a API token login in to https://id.atlassian.com/manage/api-tokens
Click Create API Token, then Enter a label for you token and click Create.
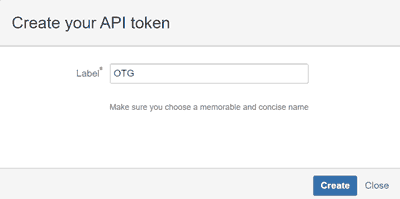
Click Copy to Clipboard and paste it on your script or save it in a file.
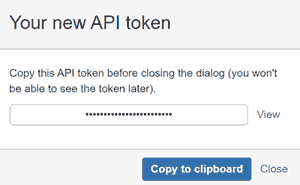
Now that you have the API token, to use it in your script first you create a string of the form:
email:api_token
Then you Base64 encode that string and prefix it with the string "Basic"
. Pass this stringin request header with field name as Authorization. Here is an example of how to do this in Python.
import base64 cred = "Basic " + base64.b64encode(b'myemail@otg.com:pQcIDzMucUS76VBUmwzp2012').decode("utf-8") headers = { "Accept": "application/json", "Content-Type": "application/json", "Authorization": cred }
Python program to get all issues in a Jira project
Here is a simple Python script that put it all together to get all issues from a Jira project.
import requests import json import base64 # Base encode email and api token cred = "Basic " + base64.b64encode(b'myemail@otg.com:pQcIDzMucUS76VBUmwzp2012').decode("utf-8") # Set header parameters headers = { "Accept": "application/json", "Content-Type": "application/json", "Authorization" : cred } # Enter your project key here projectKey = "PKY" # Update your site url url = "https://mysite.atlassian.net/rest/api/3/search?jql=project=" + projectKey # Send request and get response response = requests.request( "GET", url, headers=headers ) # Decode Json string to Python json_data = json.loads(response.text) # Display issues for item in json_data["issues"]: print(item["id"] + "\t" + item["key"] + "\t" + item["fields"]["issuetype"]["name"] + "\t" + item["fields"]["created"]+ "\t" + item["fields"]["creator"]["displayName"] + "\t" + item["fields"]["status"]["name"] + "\t" + item["fields"]["summary"] + "\t" )
Note: Remember to update the above script with your own Email, API token, Project key and site url.
REST APIs for Jira Cloud and Jira Server are similar and they can be used to automate and script interactions with Jira and for integrating Jira with other applications.