In this Windows 10 IoT project for Raspberry Pi, we are going to create a Wifi Connection status indicator. The whole idea of this project is to have an LED light that indicates whether your Windows IoT device has connected to a specified Wifi network or not. If the LED is off it means that your WiFi network is not available. Flashing LED means the WiFi network is available and a connection attempt is being made. If the LED light is steady ON it means connection has been made.
We will create the app to check the WiFi connection status in VB.NET. This app will will run as background service in your Raspberry Pi running Windows 10 IoT.
Hardware requirements
- Raspberry Pi 2 or 3 with Windows 10 IoT Core installed
- LED light
- Resistor 220Ω (or higher upto 1KΩ)
- Breadboard
- Connector wires
Wiring the LED
Connect the negative end of LED to GPIO 5 and positive end to 3.3V pin using the resistor. Check this link for detailed instructions. Connecting LED to Raspberry Pi GPIO pins
Building the app
Create a new Visual Studio Project in Visual Basic using the template Background Application (IoT). For your app to access the Wi-Fi adapter, the wifiControl DeviceCapability must be added to the AppX manifest. To do this add the following line in manifest file Package.appx.
<DeviceCapability Name="wifiControl" />
All your app code is written inside the StartupTask
class. The following are the main functions.
- initGPIO - Initialize the GPIO Controller.
The initGPIO function gets the GPIO controller, opens the GPIO pin 5 and writes a low value to the pin and also sets the Pin for output. This function is executed only once at the beginnig of the program. - wifiInit - Access the WiFi adapter.
The wifiInit function requests access to the WiFi adapters in your device, gets the first adapter (in case you have multiple wifi adapater). It will then scan the adapter for available Wifi networks. This function is executed only once at the beginnig of the program - wifiConnect - Connect to the WiFi network.WifiConnect function scans through list of available networks to check if the wifi network that you want to connect exists and tries to make a connection to that network using the specified password credentials. This function is executed whenever the wifi connection status changes to not connected (
wifiConnected = False
) - SetWifiConnectionStatus - Set Wifi connection status. This function is executed periodically to check if the WiFi connection has been established. It will set the variable
wifiConnected
to either True or False - WiFiPollTimer_tick - Periodic Timer to check the connection status and make the LED on, off or blink. The code inside this function is executed every 2 seconds.
The program also uses the following constants
MYWIFI
A string constant to specify the name of your WiFi network.PASSWORD
Password for your Wifi network.INTERVAL
The interval in seconds between connection status checks. You may change this value according to your requirements.LED_PIN
The GPIO pin to which the LED is connected.
Source Code
Here is the full source code of StartupTask class.
Imports Windows.ApplicationModel.Background Imports Windows.Devices.Gpio Imports Windows.System.Threading Imports Windows.Devices.WiFi Imports Windows.Networking.Connectivity Imports Windows.Security.Credentials Public NotInheritable Class StartupTask Implements IBackgroundTask Const LED_PIN As Short = 5 'GPIO Pin(5) = Physical Pin(29) = WiringPi Pin (21) Const INTERVAL As Short = 2 'Interval in seconds Const MYWIFI As String = "MyWiFiNetwork-SSID" ' Your wifi ssid Const MYPASSWORD As String = "password" 'Your wifi password Private gpio Private pin As GpioPin Private pinValue As GpioPinValue Private BlinkTimer As ThreadPoolTimer Private cancelled As Boolean = False Private deferral As BackgroundTaskDeferral = Nothing Private FirstAdapter As WiFiAdapter Private wifiNetworks As WiFiNetworkReport Private mywifinetwork As WiFiAvailableNetwork Private networkfound As Boolean = False Private connectedProfile As ConnectionProfile Private wifiConnected As Boolean = False Public Sub Run(taskInstance As IBackgroundTaskInstance) Implements IBackgroundTask.Run 'Handler for cancelled background task AddHandler taskInstance.Canceled, New BackgroundTaskCanceledEventHandler(AddressOf onCanceled) 'Continue background task execution deferral = taskInstance.GetDeferral() 'Initialize GPIO initGPIO() 'Initialize Wifi wifiInit() 'Create a periodic timer BlinkTimer = ThreadPoolTimer.CreatePeriodicTimer(New TimerElapsedHandler(AddressOf WiFiPollTimer_tick), TimeSpan.FromSeconds(INTERVAL)) End Sub Private Async Sub wifiInit() Dim wifiaccess As WiFiAccessStatus 'Request access to the wifi adapters wifiaccess = Await WiFiAdapter.RequestAccessAsync() If wifiaccess = WiFiAccessStatus.Allowed Then 'Find all WiFi adapters Dim result = Await WiFiAdapter.FindAllAdaptersAsync() If (result.Count >= 1) Then FirstAdapter = result(0) 'Scan for available Wifi networks Await FirstAdapter.ScanAsync wifiNetworks = FirstAdapter.NetworkReport End If End If End Sub Private Async Sub wifiConnect(wifinetwork As WiFiAvailableNetwork) Dim result As WiFiConnectionResult Dim credential As New PasswordCredential credential.Password = MYPASSWORD 'FirstAdapter.Disconnect() result = Await FirstAdapter.ConnectAsync(wifinetwork, WiFiReconnectionKind.Automatic, credential) If result.ConnectionStatus = WiFiConnectionStatus.Success Then Debug.WriteLine("Connected Now... ") wifiConnected = True End If End Sub Private Async Sub SetWifiConnectionStatus(ssid As String) connectedProfile = Await FirstAdapter.NetworkAdapter.GetConnectedProfileAsync() If Not (connectedProfile Is Nothing) AndAlso connectedProfile.GetNetworkNames(0) = ssid Then Debug.WriteLine("Connected to Wifi") wifiConnected = True Else wifiConnected = False Debug.WriteLine("Disconnected..") End If End Sub Private Sub onCanceled(sender As IBackgroundTaskInstance, reason As BackgroundTaskCancellationReason) cancelled = True End Sub Sub WiFiPollTimer_tick(state As Object) If Not cancelled AndAlso Not (FirstAdapter Is Nothing) Then If wifiNetworks Is Nothing Then 'If there are no available networks turn LED OFF pinValue = GpioPinValue.High pin.Write(pinValue) networkfound = False Else If networkfound = False Then 'If there are available networks in range For Each AvailableNetwork In wifiNetworks.AvailableNetworks 'if wifi network is available in range If AvailableNetwork.Ssid = MYWIFI Then networkfound = True mywifinetwork = AvailableNetwork End If Next Else SetWifiConnectionStatus(mywifinetwork.Ssid) If wifiConnected = False Then wifiConnect(mywifinetwork) If (pinValue = GpioPinValue.High) Then pinValue = GpioPinValue.Low Else pinValue = GpioPinValue.High End If pin.Write(pinValue) Else Debug.WriteLine(mywifinetwork.Ssid) If pinValue <> GpioPinValue.Low Then pinValue = GpioPinValue.Low pin.Write(pinValue) End If End If End If End If Else 'if the task is cancelled 'Stop the timer and finish execution BlinkTimer.Cancel() deferral.Complete() End If End Sub Private Sub initGPIO() 'Get the default GPIO controller gpio = GpioController.GetDefault() If Not gpio Is Nothing Then 'Open a connection to the GPIO Pin pin = gpio.openpin(LED_PIN) pinValue = GpioPinValue.High 'Write Pin value low to turn OFF LED pin.Write(pinValue) 'Set the pin for output pin.SetDriveMode(GpioPinDriveMode.Output) End If End Sub End Class
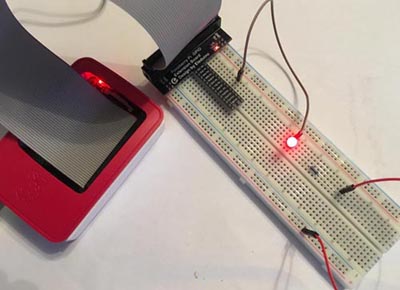